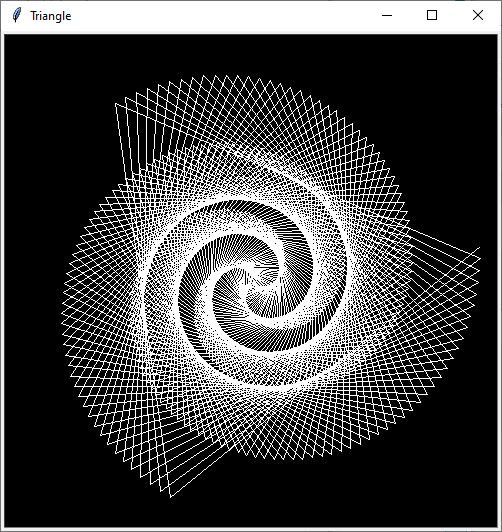
Python Turtle
Since I found Python Turtle on my desktop the other day, and since there’s not much leisure activities to choose from right now because of Corona, I’ve been looking into Turtle again.
Da ich letztens Python Turtle auf meinem Desktop wiedergefunden habe und da es wegen Corona gerade nicht viel Auswahl an Freizeitaktivitäten gibt, habe ich mich seit langem mal wieder mit Turtle beschäftigt.
To get started, it is always a good idea to play around with the program first. That’s exactly what I did, which resulted in the following:
Als Einstieg bietet sich natürlich immer an, erst einmal mit dem Programm herumzuspielen. Eben das habe ich auch gemacht, woraus folgendes entstanden ist:
Spirograph
This is basically a programmed version of a spirograph. Therefore, the colored circles rotate around the center.
Das ist im Prinzip eine programmierte Version eines Spirographen. Demnach rotierten die bunten Kreise um das Zentrum.
First, all the required packages, or parts of packages, are imported. That would be turtle first, of course, since we want to paint something. It doesn’t matter if you use import turtle
or from turtle import *
, because this script is kept simple. from turtle import *
imports all modules available for Turtle, which are not needed here yet.
Additionally we import the module cycle
from the package itertools
. The module will be used later for the colour change.
Zunächst werden alle benötigten Pakete, beziehungsweise Teile von Paketen importiert. Das wäre dann natürlich erst einmal Turtle, wir möchten ja etwas malen. Hier ist es egal, ob ihr import turtle
oder from turtle import *
verwendet, da dieses Skript einfach gehalten ist. from turtle import *
importiert alle für Turtle erhältlichen Module, die hier aber nicht benötigt werden.
Zusätzlich importieren wir noch das Modul cycle
aus dem Paket itertools
. Das Modul wird später zum Farbwechsel verwendet.
import turtle
from turtle import *
from itertools import cycle
Now we come to the actual setup, that is how the window will look like afterwards, and to the settings of the Turtle, which is the pen in this case.
turtle.setup()
sets the size of the window. turtle.title()
changes the title that appears in the top left of the window header, whereas turtle.bgcolor()
sets the background color of the window. The turtle’s settings regarding the size of the pen and the speed at which the turtle moves are defined with turtle.pensize()
and turtle.speed()
.
Jetzt kommen wir zum eigentlichen Setup, also dazu wie das Fenster nachher aussehen wird, und zu den Einstellungen der Turtle, also dem Stift in diesem Fall.
Mit turtle.setup()
wird die Größe des Fensters bestimmt. turtle.title()
verändert den Titel, der oben links in der Kopfzeile des Fensters erscheint, wohingegen turtle.bgcolor()
die Hintergrundfarbe des Fensters festlegt. Die Einstellungen der Turtle bezüglich der Größe des Stiftes und der Geschwindigkeit, mit der sich die Turtle bewegt, werden mit turtle.pensize()
und turtle.speed()
definiert.
turtle.setup(500, 500)
turtle.title("Spigrograph")
turtle.bgcolor("black")
turtle.pensize(1)
turtle.speed(0)
Next, we define a variable which we define a list of colors in which the circles will later be drawn in sequence with the help of the module cycle
.
Als nächstes definieren wir eine Variable, hinter der wir mit Hilfe des Moduls cycle
eine Liste an Farben definieren, in denen später die Kreise der Reihe nach gezeichnet werden.
colours = cycle(['cyan', 'blue', 'green', 'yellow', 'orange', 'red', 'magenta', 'white'])
Now we finally get to the circles. Therefore, we write a loop so that we only have to write everything once. In Python you start a loop with for i in range(x):
, which is then executed x
times. The content of the loop defines the size and color of the circle and that the turtle must rotate after each circle.
In this case, the loop is executed 37 times so that the circle closes. Each of the 37 circles drawn by turtle.circle(50)
has a diameter of 50. After that, the turtle turns 10 degrees to the left by turtle.left(10)
and changes the colour to the next colour of the variable colours
we defined earlier with the help of the cycle
module by turtle.color(next(colours))
.
Jetzt geht es endlich an die Kreise. Hierfür schreiben wir eine Schleife, damit wir alles nur einmal schreiben müssen. In Python beginnt man eine Schleife mit for i in range(x):
, die dann x
-mal ausgeführt wird. Der Inhalt der Schleife definiert den die Größe und Farbe des Kreises und dass sich die Turtle drehen muss.
In diesem Fall wird die Schleife 37-mal ausgeführt, sodass sich der Kreis schließt. Dabei hat jeder der 37 Kreise, die durch turtle.circle(50)
gezeichnet werden, den Durchmesser 50. Danach dreht sich die Turtle, durch turtle.left(10)
um 10 Grad nach links und wechselt mit Hilfe des Moduls cycle
die Farbe zu nächsten Farbe der von uns vorhin definierten Variable colours
, durch turtle.color(next(colours))
.
for i in range(37):
turtle.circle(50)
turtle.left(10)
turtle.color(next(colours))
Finally, we use turtle.hideturtle()
, so that the turtle is not visible after drawing and does not disturb the overall image.
Zum Schluss nutzen wir turtle.hideturtle()
, sodass die Turtle nach dem Zeichnen nicht mehr zu sehen ist und das Gesamtbild nicht stört.
turtle.hideturtle()
Finished Code / Fertiger Code
import turtle
from turtle import *
from itertools import cycle
turtle.setup(500, 500)
turtle.title("Spigrograph")
turtle.bgcolor("black")
turtle.pensize(1)
turtle.speed(0)
colours = cycle(['cyan', 'blue', 'green', 'yellow', 'orange', 'red', 'magenta', 'white'])
for i in range(37):
turtle.circle(50)
turtle.left(10)
turtle.color(next(colours))
turtle.hideturtle()
Hexagon
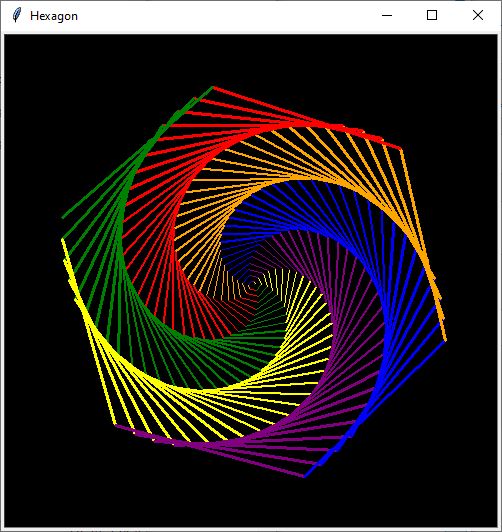
This drawing is actually a spiral in hexagonal shape. This means, that the pen needs to draw everything in one stroke.
Diese Zeichnung ist eigentlich eine Spirale in sechseckiger Form. Das heißt, dass der Stift alles in einem Zug malt.
First we import again the same packages as above and define the size and title of the windoe. As well as the background colour and the variable for the different colours.
Zuerst importieren wir wieder sie selben Pakete wie oben und definieren die Größe und den Titel des Fensters. Genauso wie die Hintergrundfarbe und die Variable für die unterschiedlichen Farben.
import turtle
from turtle import *
from itertools import cycle
turtle.setup(500, 500)
colours = cycle(['red', 'green', 'yellow', 'purple', 'blue','orange'])
turtle.title("Hexagon")
turtle.bgcolor('black')
Let’s go directly to the loop. This time the number of repetitions depends on the size of the hexagon. Within the loop, turtle.color(next(colours))
is used again to cause the turtle to change its colour after each line.
New, however, is the variable that determines how far the turtle moves and the calculation of the size of the pen. For this you have to know that i
counts the number of repetitions, meaning that i
increases by 1 per repetition, which in this case is 200 times. i
therefore adopts every value from 1 to 200 once. Since the hexagon should grow, each new edge must be longer than the previous one, which is why i
simultaneously defines the length of the stroke through turtle.forward(i)
.
Since the size of the pencil should increase proportional to the length of the individual edges, the size x
must be calculated dependent on length i
. This results in the formula x = i/100+ 1
. Here, the length of the edge is divided by 100 and added to 1. The number by which the length of the edge is divided depends on how fast the size of the pen is to increase. Adding 1 is necessary because otherwise not all edges are visible if the width of the pen is too thin. The calculated size is inserted into turtle.pensize(x)
.
Kommen wir also direkt zur Schleife. Diese Mal ist die Anzahl an Wiederholungen abhängig davon, wie groß das Hexagon werden soll. Innerhalb der Schliefe wird wiederum mit turtle.color(next(colours))
festgelegt, dass die Turtle nach jeder Kante die Farbe wechselt.
Neu ist allerdings die Variable, die bestimmt, wie weit sich die Turtle bewegt und die Berechnung der Dicke des Stiftes. Hierfür muss man wissen, das i
die Anzahl an Wiederholungen zählt, also dass i
pro Durchlauf um 1 zunimmt und das eben in diesem Fall 200-mal. i
nimmt dementsprechend jeden Wert von 1 bis 200 einmal an. Da das Hexagon wachsen soll, muss jede neue Kante länger sein als die vorherige, weswegen i
gleichzeitig durch turtle.forward(i)
die Länge des Striches definiert.
Da die Dicke des Stiftes ebenso, proportional zur Länge der einzelnen Kanten, zunehmen soll, muss sie Dicke x
in Abhängigkeit von der Länge i
berechnet werden. Dadurch kommt es zu der Formel x = i/100+ 1
. Hierbei wird die Länge der Kante durch 100 geteilt und zu 1 addiert. Die Zahl, durch die die Länge der Kante geteilt wird, ist dabei abhängig davon, wie schnell die Dicke des Stifte zunehmen soll. Das Addieren um 1 ist allerdings nötig, da sonst nicht alle Kanten sichtbar sind wenn die Dicke des Stiftes zu dünn ist. Eingesetzt wird die Errechnete Dicke in turtle.pensize(x)
.
for i in range(200):
turtle.color(next(colours))
x = i/100 + 1
turtle.pensize(x)
turtle.forward(i)
turtle.left(59)
Last but not least, after drawing, the turtle is hidden again with turtle.hideturtle()
.
Zu guter Letzt wird die Turtle nach dem Zeichnen wieder mit turtle.hideturtle()
versteckt.
turtle.hideturtle()
Finished Code / Fertiger Code
import turtle
from turtle import *
from itertools import cycle
turtle.setup(500, 500)
colours = cycle(['red', 'green', 'yellow', 'purple', 'blue','orange'])
turtle.title("Hexagon")
turtle.bgcolor('black')
for i in range(200):
turtle.color(next(colours))
x = i/100 + 1
turtle.pensize(x)
turtle.forward(i)
turtle.left(59)
turtle.hideturtle()
Triangle / Dreieck
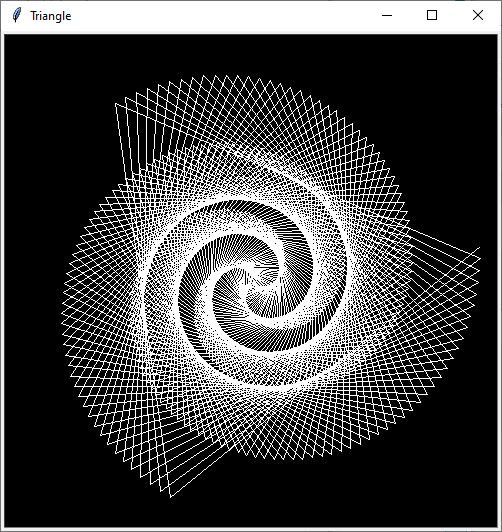
This script is a variation of the hexagon. However, here I have changed the angle so that it becomes a spiral in triangular form.
Dieses Skript ist eine Variation des Hexagons. Allerdings habe ich hier den Winkel so verändert, dass daraus eine Spirale in dreieckiger Form daraus wird.
Again, everything necessary is imported first and the setup gets set:
Auch hier wird zuerst wieder alles Nötige importiert und das Setup festgelegt:
import turtle
from turtle import *
from itertools import cycle
turtle.setup(500, 500)
colours = cycle(['white'])
turtle.title("Triangle")
turtle.bgcolor('black')
turtle.speed(0)
In the loop, the colour change is set up again. In this case, I set the size of the pen to 0.5, although this can also be dynamized, too. As with the hexagon, the number of repetitions also defines the length of each edge.
However, I have increased the angle around which the turtle rotates after drawing each edge. Since a perfect triangle has an exterior angle of 120 degrees at each corner, the angle must be slightly larger than 120, because we don’t want only one triangle to be drawn, but many.
In der Schleife wird der Farbwechsel wieder eingerichtet. In diesem Fall habe ich die Dicke des Stiftes auf 0,5 festgelegt, welche allerdings auch dynamisiert werden kann. Ebenso wie beim Hexagon definiert die Anzahl an Wiederholungen auch die Länge der einzelnen Kanten.
Den Winkel, um den sich die Turtle nach dem Zeichnen jeder Kante dreht, habe ich allerdings vergrößert. Da ein perfektes Dreieck bei jeder Ecke einen Außenwinkel von 120 Grad hat, wir aber ja nicht wollen, dass nur ein Dreieck gezeichnet wird, sondern viele, muss der Winkel etwas größer als 120 sein.
for i in range(400):
turtle.color(next(colours))
turtle.pensize(0.5)
turtle.forward(i)
turtle.left(121)
Finally, again, we don’t want the turtle to be visible after drawing.
Zum Schluss wollen wir wieder nicht, dass die Turtle nach dem Zeichnen noch sichtbar ist.
turtle.hideturtle()
Finished Code / Fertiger Code
import turtle
from turtle import *
from itertools import cycle
turtle.setup(500, 500)
colours = cycle(['white'])
turtle.title("Triangle")
turtle.bgcolor('black')
turtle.speed(0)
for i in range(400):
turtle.color(next(colours))
turtle.pensize(0.5)
turtle.forward(i)
turtle.left(121)
turtle.hideturtle()
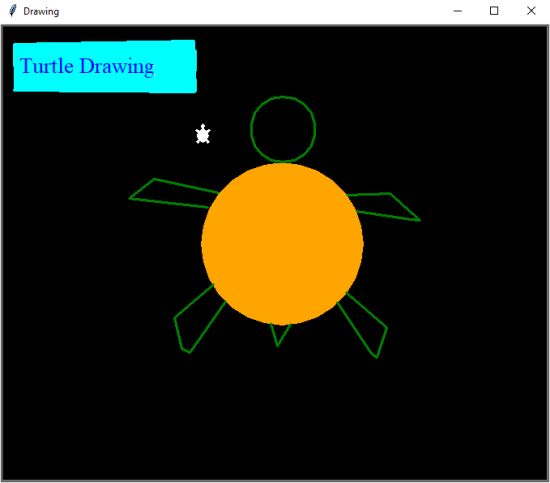

One Comment
Pingback: